SpringBoot 入力項目
Thymeleafでテキスト入力以外の入力項目をやります。テキスト入力はThymeleaf基本を参照してください。全体
UserModel.java
package spring.test;
import java.util.List;
public class UserModel {
private String sel1;
private String[] sel2;
private boolean chk1;
private String rdo1;
private String hdn;
private List<DetailModel> details;
public String toString() {
StringBuilder stb = new StringBuilder();
stb.append("sel1:" + sel1 + System.lineSeparator());
for (String str : sel2) {
stb.append("sel2:" + str + System.lineSeparator());
}
stb.append("chk1:" + chk1 + System.lineSeparator());
stb.append("rdo1:" + rdo1 + System.lineSeparator());
stb.append("hdn:" + hdn + System.lineSeparator());
for (DetailModel model : details) {
stb.append("ID:" + model.getId() + " VAL:" + model.getVal() + System.lineSeparator());
}
return stb.toString();
}
public String getSel1() {
return sel1;
}
public void setSel1(String sel1) {
this.sel1 = sel1;
}
public String[] getSel2() {
return sel2;
}
public void setSel2(String[] sel2) {
this.sel2 = sel2;
}
public boolean isChk1() {
return chk1;
}
public void setChk1(boolean chk1) {
this.chk1 = chk1;
}
public String getRdo1() {
return rdo1;
}
public void setRdo1(String rdo1) {
this.rdo1 = rdo1;
}
public String getHdn() {
return hdn;
}
public void setHdn(String hdn) {
this.hdn = hdn;
}
public List<DetailModel> getDetails() {
return details;
}
public void setDetails(List<DetailModel> details) {
this.details = details;
}
}
DetailModel.java
package spring.test;
public class DetailModel {
String id;
String val;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVal() {
return val;
}
public void setVal(String val) {
this.val = val;
}
}
LoginControler.java
package spring.test.controller;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.servlet.ModelAndView;
import spring.test.UserModel;
import spring.test.DetailModel;
@Controller
@RequestMapping(value = "/login")
public class LoginControler {
private List<DetailModel> getDetails() {
List<DetailModel> models = new ArrayList<>();
DetailModel model1 = new DetailModel();
DetailModel model2 = new DetailModel();
model1.setId("a");
model1.setVal("aaa");
model2.setId("b");
model2.setVal("bbb");
models.add(model1);
models.add(model2);
return models;
}
public void setMap(Model model) {
LinkedHashMap<String, String> map = new LinkedHashMap<>();
map.put("A", "その1");
map.put("B", "その2");
model.addAttribute("map", map);
}
@RequestMapping(method = RequestMethod.GET)
public ModelAndView loginGet(@ModelAttribute("UM") UserModel userModel, Model model) {
ModelAndView mv = new ModelAndView("login");
userModel.setSel1("B");
String[] strs = { "B" };
userModel.setSel2(strs);
userModel.setChk1(true);
userModel.setRdo1("B");
userModel.setDetails(getDetails());
userModel.setHdn("ABC");
setMap(model);
return mv;
}
@RequestMapping(method = RequestMethod.POST)
public ModelAndView loginPost(@ModelAttribute("UM") UserModel userModel, Model model) {
ModelAndView mv = new ModelAndView("login");
System.out.println(userModel.toString());
return mv;
}
}
login.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>ログイン</title>
<style type="text/css">
table {
border-collapse: collapse;
border: solid 1px;
}
td {
border: solid 1px;
}
th {
background-color: gray;
border: solid 1px;
}
h4 {
margin: 5px;
}
</style>
</head>
<body>
<form th:action="@{/login}" method="post" th:object="${UM}">
<div>
<h4>ドロップダウン</h4>
<select th:field="*{sel1}">
<option th:each="item : ${map}" th:value="${item.key}" th:text="${item.value}" />
</select>
</div>
<div>
<h4>セレクトボックス</h4>
<select th:field="*{sel2}" multiple="multiple" size="2">
<option th:each="item : ${map}" th:value="${item.key}" th:text="${item.value}" />
</select>
</div>
<div>
<h4>チェックボックス</h4>
<label th:for="${#ids.next('chk1')}" th:text="チェック">check</label>
<input type="checkbox" th:field="*{chk1}" />
</div>
<div>
<h4>ラジオボタン</h4>
<ul>
<li th:each="item : ${map}">
<input type="radio" th:field="*{rdo1}" th:value="${item.key}" />
<label th:for="${#ids.prev('rdo1')}" th:text="${item.value}">ラジオ</label>
</li>
</ul>
</div>
<div>
<h4>Hidden</h4>
<input type="hidden" th:field="*{hdn}" />
</div>
\ <h4>一覧</h4>
<table>
<tr>
<th>ID</th>
<th>VAL</th>
</tr>
<tr th:each="detail : *{details}">
<td th:text="${detail.id}"></td>
<td th:text="${detail.val}"></td>
</tr>
</table>
<h4>一覧(入力できる)</h4>
<table>
<tr>
<th>ID</th>
<th>VAL</th>
</tr>
<tr th:each="detail, loop : *{details}">
<td><input th:field="*{details[__${loop.index}__].id}" /></td>
<td><input th:field="*{details[__${loop.index}__].val}" /></td>
</tr>
</table>
<BR />
<input type="submit" value="ログイン" />
</form>
</body>
</html>
ファイル構成
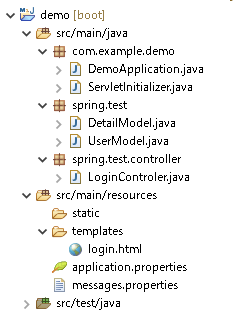
実行結果(画面)
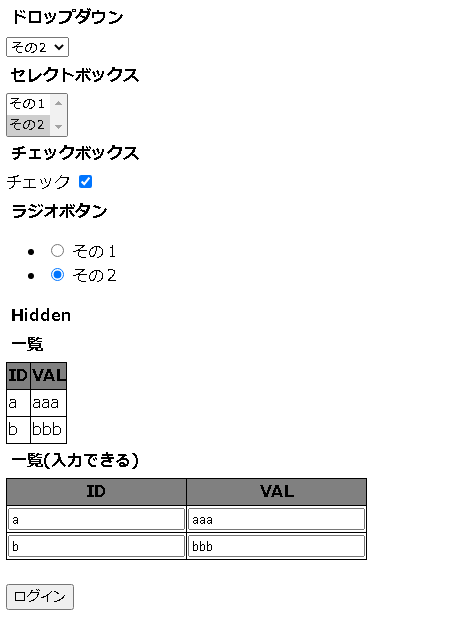
実行結果(コンソール)
sel1:B
sel2:B
chk1:true
rdo1:B
hdn:ABC
ID:a VAL:aaa
ID:b VAL:bbb
出力されるHtml
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>ログイン</title>
<style type="text/css">
table {
border-collapse: collapse;
border: solid 1px;
}
td {
border: solid 1px;
}
th {
background-color: gray;
border: solid 1px;
}
h4 {
margin: 5px;
}
</style>
</head>
<body>
<form action="/login" method="post">
<div>
<h4>ドロップダウン</h4>
<select id="sel1" name="sel1">
<option value="A" >その1</option>
<option value="B" selected="selected" >その2</option>
</select>
</div>
<div>
<h4>セレクトボックス</h4>
<input type="hidden" name="_sel2" value="1"/><select multiple="multiple" size="2" id="sel2" name="sel2">
<option value="A" >その1</option>
<option value="B" selected="selected" >その2</option>
</select>
</div>
<div>
<h4>チェックボックス</h4>
<label for="chk11">チェック</label>
<input type="checkbox" id="chk11" name="chk1" value="true" checked="checked" /><input type="hidden" name="_chk1" value="on"/>
</div>
<div>
<h4>ラジオボタン</h4>
<ul>
<li>
<input type="radio" value="A" id="rdo11" name="rdo1" />
<label for="rdo11">その1</label>
</li>
<li>
<input type="radio" value="B" id="rdo12" name="rdo1" checked="checked" />
<label for="rdo12">その2</label>
</li>
</ul>
</div>
<div>
<h4>Hidden</h4>
<input type="hidden" id="hdn" name="hdn" value="ABC" />
</div>
\ <h4>一覧</h4>
<table>
<tr>
<th>ID</th>
<th>VAL</th>
</tr>
<tr>
<td>a</td>
<td>aaa</td>
</tr>
<tr>
<td>b</td>
<td>bbb</td>
</tr>
</table>
<h4>一覧(入力できる)</h4>
<table>
<tr>
<th>ID</th>
<th>VAL</th>
</tr>
<tr>
<td><input id="details0.id" name="details[0].id" value="a" /></td>
<td><input id="details0.val" name="details[0].val" value="aaa" /></td>
</tr>
<tr>
<td><input id="details1.id" name="details[1].id" value="b" /></td>
<td><input id="details1.val" name="details[1].val" value="bbb" /></td>
</tr>
</table>
<BR />
<input type="submit" value="ログイン" />
</form>
</body>
</html>
ページのトップへ戻る