SpringBoot Thymeleaf 条件分岐
今回はThymeleafの条件分岐をやります。環境設定等はこちらを参照下さい。単純なThymeleafの画面はこちらを参照下さい。
ソース
UserModel.java
package spring.test;
import java.util.ArrayList;
import java.util.List;
public class UserModel {
boolean booTrue = true;
boolean booFalse = false;
List<String> aryNull = null;
List<String> aryZero = new ArrayList<>();
List<String> aryOne = new ArrayList<>();
String id;
int number;
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
public boolean isBooTrue() {
return booTrue;
}
public void setBooTrue(boolean booTrue) {
this.booTrue = booTrue;
}
public boolean isBooFalse() {
return booFalse;
}
public void setBooFalse(boolean booFalse) {
this.booFalse = booFalse;
}
public List<String> getAryNull() {
return aryNull;
}
public void setAryNull(List<String> aryNull) {
this.aryNull = aryNull;
}
public List<String> getAryZero() {
return aryZero;
}
public void setAryZero(List<String> aryZero) {
this.aryZero = aryZero;
}
public List<String> getAryOne() {
return aryOne;
}
public void setAryOne(List<String> aryOne) {
this.aryOne = aryOne;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
}
LoginControler.java
package spring.test.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import spring.test.UserModel;
@Controller
@RequestMapping(value = "/login")
public class LoginControler {
@RequestMapping(method = RequestMethod.GET)
public String loginGet(@ModelAttribute("UM") UserModel userModel) {
userModel.setId("aaa");
return "login";
}
@RequestMapping(method = RequestMethod.POST)
public String loginPost(@ModelAttribute("UM") UserModel userModel) {
return "login";
}
}
login.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>ログイン</title>
</head>
<body>
<form th:action="@{/login}" method="post" th:object="${UM}">
ID: <input type="text" th:field="*{id}" /><br />NUMBER:<input
type="text" th:field="*{number}" /> <br /> <input type="submit"
value="ログイン" /> <br />
<div th:if="*{id} eq 'aaa'">*{id} eq 'aaa'</div>
<div th:if="*{number} gt 0">*{number} gt 0</div>
<div th:if="*{booTrue}">th:if="*{booTrue}</div>
<div th:if="*{booFalse}">th:if="*{booFalse}</div>
<div th:unless="*{booTrue}">th:unless="*{booTrue}</div>
<div th:unless="*{booFalse}">th:unless="*{booFalse}</div>
<div th:if="*{aryNull}">aryNull</div>
<div th:if="*{aryZero}">aryZero</div>
<div th:if="*{aryOne}">aryOne</div>
<div th:switch="*{id}">
<p th:case="aaa">aaa</p>
<p th:case="bbb">bbb</p>
<p th:case="*">その他</p>
</div>
</form>
</body>
</html>
実行イメージ
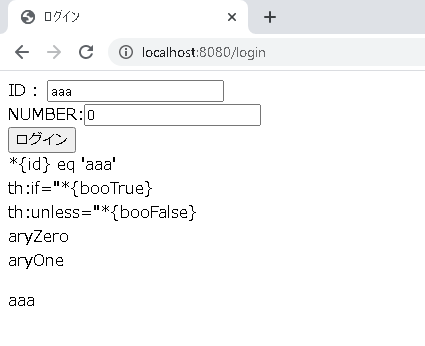
比較演算子
比較演算子 | 意味 |
---|---|
eq | == |
neq | != |
gt | > |
lt | < |
ge | >= |
le | <= |
and | AND |
or | OR |
not | NOT |
メモ
単純な条件分岐を行う場合は th:if を使用します。th:if の not 条件の場合 th:unless を使用します。
switchを行いたい場合、th:switch を使用します。条件に当てはまらない場合の処理はth:case="*" に書きます。
ページのトップへ戻る