Angular テンプレート駆動フォーム
今回は テンプレート駆動フォーム をやります。テンプレート駆動フォームはリアクティブフォームと比べて単純な画面作成時に利用します。
ロジック定義より画面定義側で色々やる感じです。
Angular CLIの続きからやるので、環境がない場合、そちらを参考に環境構築してください。
テキスト入力
formをngSubmitイベントで送信します。テンプレート変数(#fm4="ngForm")を定義し、引数に指定することで処理側に入力値を送信できます。ngModelが付いていないnm5は送信されません。
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template:
`
<form #fm4="ngForm" (ngSubmit)="onSubmit(fm4)" >
<input type='text' name="nm3" ngModel/>
<input type='text' [(ngModel)]="message4" required name="nm4" #nmMdl4="ngModel" />
<input type='text' name="nm5"/>
<div [hidden]="!nmMdl4.dirty">
<h1>入力:{{message4}}</h1>
</div>
<table>
<tr>
<td>値を1回も変更していない</td><td>{{nmMdl4.pristine}}</td>
</tr>
<tr>
<td>値が変更された</td><td>{{nmMdl4.dirty}}</td>
</tr>
<tr>
<td>フォーカスがあった</td><td>{{nmMdl4.touched}}</td>
</tr>
<tr>
<td>フォーカスがない</td><td>{{nmMdl4.untouched}}</td>
</tr>
<tr>
<td>値が有効</td><td>{{nmMdl4.valid}}</td>
</tr>
</table>
<button type="submit" [disabled]="!fm4.form.valid">送信</button>
</form>
`
})
export class AppComponent {
message4 = "ここをチェック";
onSubmit(fm: any) {
console.log(JSON.stringify(fm.value));
alert(JSON.stringify(fm.value));
}
}
テンプレート駆動フォームを使用する場合、 app.module.tsモジュールでFormsModuleをimportし、@NgModuleのimportsにFormsModuleを指定する必要があります。
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
以下で実行できます。
ng serve --open
実行イメージ
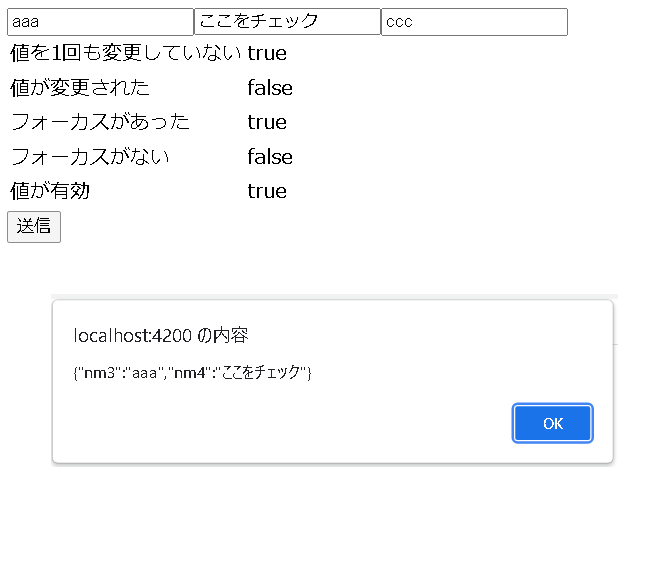
入力項目に対してチェックできること
指定 | True時の状態 |
---|---|
pristine | 値を1回も変更していない |
dirty | 値が変更された |
touched | フォーカスがあった |
untouched | フォーカスがない |
valid | 値が有効 |
form.valid | フォームすべての値が有効 |
リスト入力
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template:
`
<form #fm="ngForm" (ngSubmit)="onSubmit(fm)">
<select [(ngModel)]="message" name="cmb" >
<option *ngFor="let str of ary" value={{str}}>test_{{str}}</option>
</select>
<h1>{{message}}</h1>
<button type="submit">送信</button>
</form>
`
})
export class AppComponent {
message = "bbb";
ary = ["aaa", "bbb", "ccc"];
onSubmit(fm: any) {
console.log(JSON.stringify(fm.value) + " ---" + this.message);
alert(JSON.stringify(fm.value) + " ---" + this.message);
}
}
app.module.tsは上記から変更なし。
実行イメージ
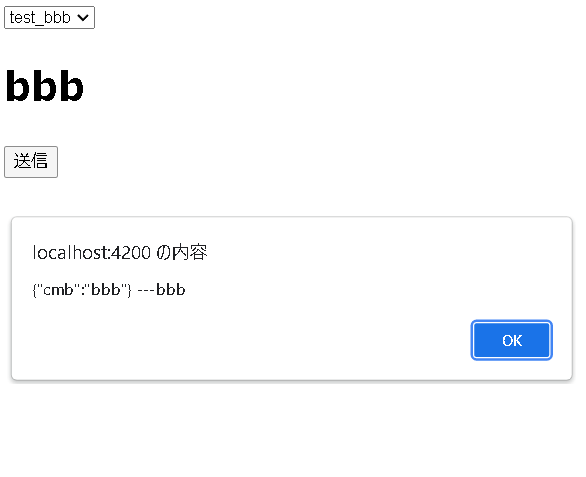
ページのトップへ戻る