Angular 親子関係
親子関係を作って、連携してみます。入力項目の続きからやるので、環境がない場合、そちらを参考に環境構築してください。
親から子に連携
src\app\app.component.ts
入力項目で作成したコンポーネントを親コンポーネントに変更。
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template:
`<input #hello (keyup)="keyupEvent(hello.value)" placeholder="ここに入力" />
<app-child [koMsg]="oyaMsg"></app-child>`
})
export class AppComponent {
oyaMsg = "ここに表示されます。";
keyupEvent(value: string) {
this.oyaMsg = value;
}
}
Angular CLIで子コンポーネントを作成。
ng generate component child
app\child\child.component.ts
@Input()で親からのデータを受け取ります。
import { Component } from '@angular/core';
import { Input } from '@angular/core';
@Component({
selector: 'app-child',
template:
`<h1>{{koMsg}}</h1>`
})
export class ChildComponent {
@Input() koMsg: String = '';
}
実行イメージ
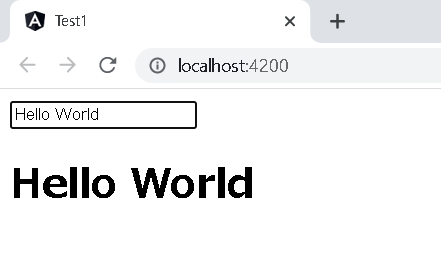
子から親に連携
src\app\app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template:
`<app-child (koOutput)="call($event)"></app-child>
<h1>{{oyaMsg}}</h1>`
})
export class AppComponent {
oyaMsg: String = "ここに表示されます。";
call(koMsg: String) {
this.oyaMsg = koMsg;
}
}
app\child\child.component.ts
@Output()で親にデータを送ります。送るときはEventEmitter送り、$eventで受け取ります。
import { Component } from '@angular/core';
import { Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template:
`<input #hello (keyup)="keyupEvent(hello.value)" placeholder="ここに入力" />`
})
export class ChildComponent {
@Output() koOutput = new EventEmitter<String>();
keyupEvent(koMsg: string) {
this.koOutput.emit(koMsg);
}
}
実行イメージ
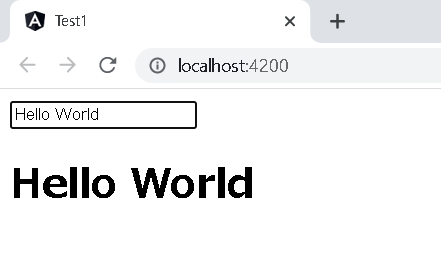
子と親の連携
今までは文字列の連携でしたが、今回はオブジェクトで連携してみます。Angular CLIでデータ連携用クラスを作成。
ng generate class Data
src\app\data.ts
export class Data {
constructor(
public key: string,
public val: string
) { }
}
src\app\app.component.ts
import { Component, OnInit } from '@angular/core';
import { Data } from './data';
@Component({
selector: 'app-root',
template:
`
<table>
<tr *ngFor="let oyaData of datas">
<app-child [koInput]="oyaData" (koOutput)="call($event)"></app-child>
</tr>
</table>
<h1>{{oyaMsg}}</h1>
`
})
export class AppComponent implements OnInit {
datas: Data[] = [];
oyaMsg: String = "ここに表示されます。";
ngOnInit(): void {
this.datas.push(new Data("key1", "val1"));
this.datas.push(new Data("key2", "val2"));
}
call(eventData: Data) {
this.oyaMsg = eventData.key + ":" + eventData.val;
this.datas.push(eventData);
}
}
child.component.ts
import { Component, EventEmitter, Input, Output } from '@angular/core';
import { Data } from '../data';
@Component({
selector: 'app-child',
template:
`
<td>{{koInput.key}}</td>
<td>{{koInput.val}}</td>
<td><button (click)="clicked(koInput)">button</button></td>
`
})
export class ChildComponent {
@Input() koInput!: Data;
@Output() koOutput = new EventEmitter<Data>();
clicked(clickData: Data) {
clickData.val += "+";
this.koOutput.emit(clickData);
}
}
実行イメージ
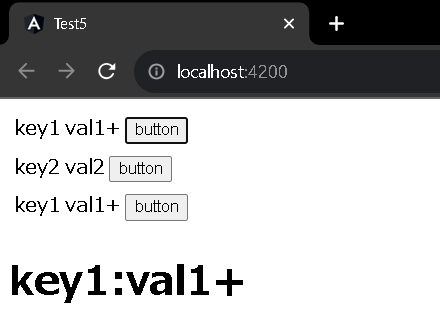
ページのトップへ戻る