Jspreadsheet CE
Jspreadsheet CEはテーブル表示のライブラリです。Jspreadsheet CEはMIT ライセンス(無償)、Jspreadsheet Proは有償になります。
テーブル表示
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
var data = [
['text1', 'aaa'],
['text2', 'bbb'],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{
type: 'text',
title: 'title1',
},
{
type: 'dropdown',
title: 'title2',
source: [
"aaa",
"bbb",
"ccc",
]
},
]
});
</script>
</body>
</html>
実行結果
スタイル
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
<style type="text/css">
.xxx {
/* 背景色 */
background-color: pink;
/* 文字色 */
color: rgb(0, 139 , 139 );
}
</style>
</head>
<body>
<div id="spreadsheet"></div>
<script>
var data = [
['text1', 'aaa'],
['text2', 'bbb'],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{
type: 'text',
title: 'title1',
width: '100px',
},
{
type: 'text',
title: 'title2',
}
],
style: {
A1: 'background-color: yellow;text-align: left;'
},
updateTable: function (el, cell, x, y, source, value, id) {
if (x == 0 && y == 1) {
cell.classList.add('xxx');
}
}
});
</script>
</body>
</html>
実行結果
読み込み専用
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
var data = [
['text1', 'aaa'],
['text2', 'bbb'],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{
type: 'text',
title: 'title1',
},
{
type: 'text',
title: 'title2',
readOnly: true,
}
],
updateTable: function (el, cell, x, y, source, value, id) {
if (x == 0 && y == 1) {
cell.classList.add('readonly');
}
}
});
</script>
</body>
</html>
実行結果
マージセル
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
var data = [
['text1', 'aaa'],
['text2', 'bbb'],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{
type: 'text',
title: 'title1',
},
{
type: 'text',
title: 'title2',
},
],
mergeCells: {
A1: [2, 1]
},
});
table.setMerge('A2', 2, 1);
// table.removeMerge('A2');
// table.destroyMerged();
</script>
</body>
</html>
実行結果
入力
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
var data = [
['text1', 'abc', 'bcd', 'cde', '2023/09/05', true, 1234],
['text2', 'abc', 'bcd', 'cde', '2023/09/06', true, 123],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{ type: 'text', title: 'text' },
{ type: 'dropdown', title: 'dropdown', width: 100,source: ["abc", "bcd", "cde"] },
{ type: 'dropdown', title: 'autocomplete', width: 120,source: ["abc", "bcd", "cde"], autocomplete: true },
{ type: 'dropdown', title: 'multiple', width: 100, source: ["abc", "bcd", "cde"], multiple: true },
{ type: 'calendar', title: 'calendar', width: 100, options: { format: 'YYYY/MM/DD' } },
{ type: 'checkbox', title: 'checkbox', width: 100, },
{ type: 'numeric', title: 'numeric', width: 100, mask:'#,##', },
],
});
</script>
</body>
</html>
実行結果
列固定
遅延読み込み
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
let columns = [];
for (let x = 0; x < 20; x++) {
columns.push({
type: 'text',
title: 'title' + x,
width: '100px',
});
}
var datas = [];
for (let y = 0; y < 500; y++) {
var data = [];
for (let x = 0; x < columns.length; x++) {
data.push("data_" + y + "_" + x);
}
datas.push(data);
}
jspreadsheet(document.getElementById('spreadsheet'), {
data: datas,
columns: columns,
tableOverflow: true,
lazyLoading: true,
tableWidth: '400px',
tableHeight: '400px',
freezeColumns: 2,
});
</script>
</body>
</html>
実行結果
行固定はJspreadsheet CEでは出来ませんでした。Jspreadsheet Proではできるみたいです。
(2023/09/19 時点)
ページネーション
検索
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<script>
let columns = [];
for (let x = 0; x < 5; x++) {
columns.push({
type: 'text',
title: 'title' + x,
width: '100px',
});
}
var datas = [];
for (let y = 0; y < 500; y++) {
var data = [];
for (let x = 0; x < columns.length; x++) {
data.push("data_" + y + "_" + x);
}
datas.push(data);
}
jspreadsheet(document.getElementById('spreadsheet'), {
data: datas,
columns: columns,
search: true,
pagination: 10,
});
</script>
</body>
</html>
実行結果
データ取得
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Jspreadsheet CE のテストページ</title>
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css"
type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
</head>
<body>
<div id="spreadsheet"></div>
<br />
<button onclick="getData()">getData</button>
<button onclick="getRowData()">getRowData</button>
<button onclick="getColumnData()">getColumnData</button>
<button onclick="getValue()">getValue</button>
<button onclick="getValueFromCoords()">getValueFromCoords</button>
<br />
<button onclick="setData()">setData</button>
<button onclick="setRowData()">setRowData</button>
<button onclick="setColumnData()">setColumnData</button>
<button onclick="setValue()">setValue</button>
<button onclick="setValueFromCoords()">setValueFromCoords</button>
<br />
<button onclick="undo()">undo</button>
<button onclick="redo()">redo</button>
<script>
var data = [
['text1', 'aaa'],
['text2', 'bbb'],
];
var table = jspreadsheet(document.getElementById('spreadsheet'), {
data: data,
columns: [
{
type: 'text',
title: 'title1',
},
{
type: 'text',
title: 'title2',
},
]
});
function getData() {
table.updateSelection(table.getCell("A1"), table.getCell("A2"), true);
alert(table.getData(true));
table.updateSelectionFromCoords(0, 0, 1, 0);
alert(table.getData(true));
alert(table.getData(false));
alert(JSON.stringify(table.getJson(false)));
}
function getRowData() {
alert(table.getRowData(0));
}
function getColumnData() {
alert(table.getColumnData(0));
}
function getValue() {
alert(table.getValue("A1"));
}
function getValueFromCoords() {
alert(table.getValueFromCoords(0, 0));
}
function setData() {
table.setData([["a", "b"], ["c", "d"]]);
}
function setRowData() {
table.setRowData(0, ["e", "f"]);
}
function setColumnData() {
table.setColumnData(0, ["g", "h"]);
}
function setValue() {
table.setValue("A1", "i", true);
}
function setValueFromCoords() {
table.setValueFromCoords(0, 0, "j", true);
}
function undo() {
table.undo();
}
function redo() {
table.redo();
}
</script>
</body>
</html>
実行結果
ライブラリ取得
URLで指定
Jspreadsheet CEを使用する場合、以下を指定します。
<script src="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/index.min.js"></script>
<script src="https://jsuites.net/v4/jsuites.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jspreadsheet-ce/dist/jspreadsheet.min.css" type="text/css" />
<link rel="stylesheet" href="https://jsuites.net/v4/jsuites.css" type="text/css" />
入手したライブラリを指定
ライブラリをローカル環境等に置いて動作させる場合は以下を取得します。- jsuites.js
- index.js
- jspreadsheet.css
- jsuites.css
https://github.com/jspreadsheet/ceにアクセスして、以下をダウンロードします。
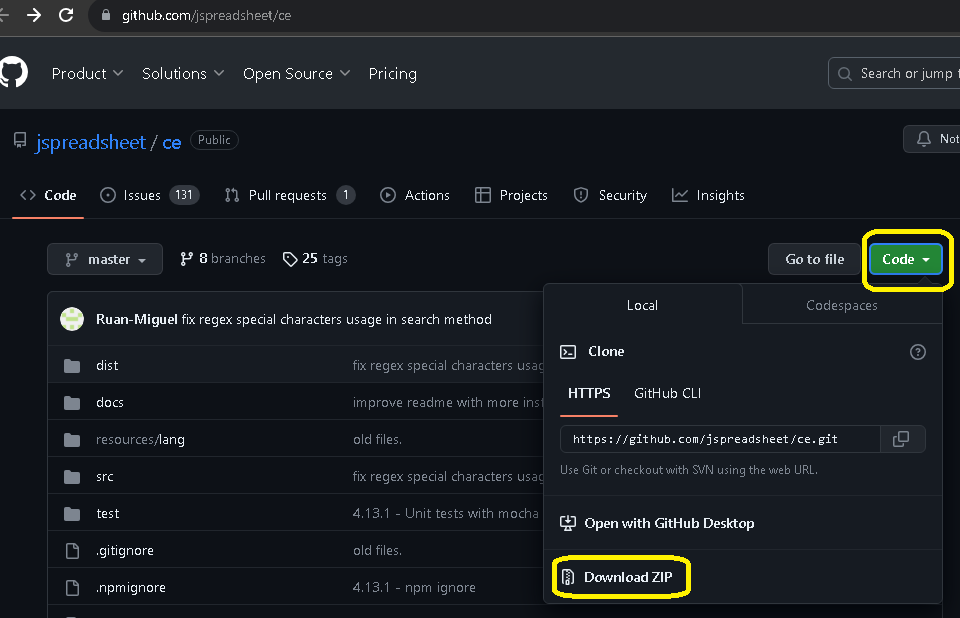
解凍してdistフォルダに対象ファイルが入っています。
https://github.com/jsuites/jsuitesからも同じようにダウンロードします。
ファイルを環境に置いた後、URLを以下のように変更します。
<script src="../jspreadsheet-ce/index.js"></script>
<script src="../jspreadsheet-ce/jsuites.js"></script>
<link rel="stylesheet" href="../jspreadsheet-ce/jspreadsheet.css" type="text/css" />
<link rel="stylesheet" href="../jspreadsheet-ce/jsuites.css" type="text/css" />
ページのトップへ戻る